Flutter SDK
This SDK has the capabilities related to device intelligence and OTL
Integration Steps
At its core, the solution functions through three straightforward steps:
- Start by installing OTL SDK with mobile application.
- call the
authenticate()
method. This initialises the SDK and makes the initiate api call. - You can then utilize our API to access user insights, aiding you in deciding the subsequent actions for your user.
Flow Diagram
This diagram shows the interactions between a merchant's mobile app and Bureau's SDK.
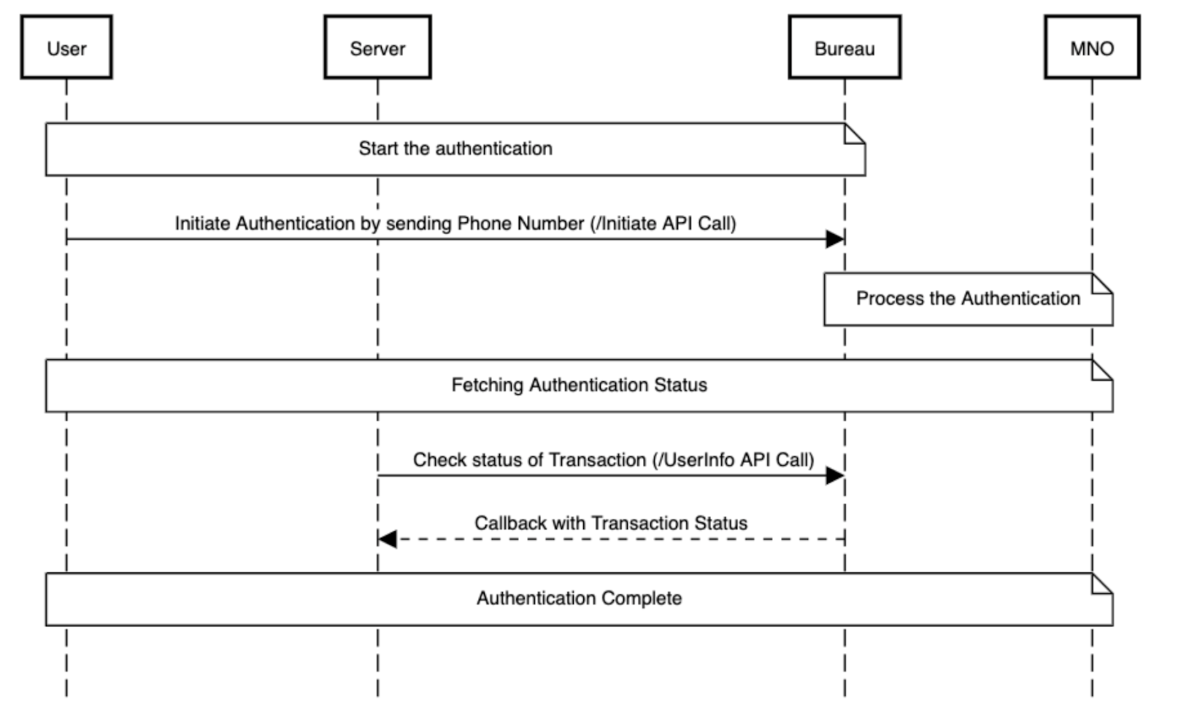
sessionId
is generated by the client backend and sent to the SDK.phoneNumber
is entered by the user- Invoke the authenticate function in SDK to make the initiate api call.
- Upon successful submission of the parameters, a callback is received in the SDK. The next steps can be taken based on the callback (success/failure).
- If the callback is successful, Invoke the Bureau's backend API
v2/auth/userinfo
to fetch user info for OTL. - Based on the user info, you can determine to login or send OTP as SMS in case of failure.
Step 1 - SDK Installation
Install onetaplogin: 1.3.8
Step 2 - Initialise SDK
Android Changes
Android outer level build.gradle changes
buildscript {
repositories {
maven {
url = uri("https://storage.googleapis.com/r8-releases/raw")
}
}
dependencies {
classpath("com.android.tools:r8:8.2.24")
}
}
Android Manifest changes
//Add the folowing call if targeting S+ devices
<activity>
android:exported="true"
</activity>
........
<application
android:usesCleartextTraffic="true"
</application>
or if you are using network config
<?xml version="1.0" encoding="utf-8"?>
<network-security-config>
<domain-config cleartextTrafficPermitted="true">
<domain includeSubdomains="false">PLEASE CONTACT BUREAU TO GET DOMAIN</domain>
</domain-config>
</network-security-config>
IOS Changes
Make the below changes to Info.plist
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
On Production
// Import package
import 'package:onetaplogin/onetaplogin.dart';
// Authenticate : phoneNumber should be starting with 91
int status = await Onetaplogin.authenticate(
clientID,
sessionId,
phoneNumber,
timeoutinMs:timeoutinMs); //we suggest atleast 4*1000 milli sec
Note
Onetaplogin.authenticate()
(OTL) is available only in Android and IOS platforms
On Sandbox
// Import package
import 'package:onetaplogin/onetaplogin.dart';
// Authenticate : phoneNumber should be starting with 91
int status = await Onetaplogin.authenticate(
clientID,
sessionId,
phoneNumber,
env : "Sandbox",
timeoutinMs:timeoutinMs); //we suggest atleast 4*1000 milli sec
The status object for authenticate method would be an integer which mean as mentioned in SDK Responses
SDK Responses
Status | Description |
---|---|
1 | AuthenticationStatus.Completed; |
-2 | AuthenticationStatus.NetworkUnavailable; |
-3 | AuthenticationStatus.OnDifferentNetwork; |
-4 | AuthenticationStatus.ExceptionOnAuthenticate; |
0(default) | AuthenticationStatus.UnknownState; |
Step 3 - Invoke API for User Info
To access insights from users for OTL, integrating with Bureau's backend API is a must for both OTL.
Please find below the link to the respective API documentation:
OTL: https://docs.bureau.id/reference/one-tap-login
- Sandbox - https://api.sandbox.bureau.id/v2/auth
- Production - https://api.bureau.id/v2/auth
Authentication
API's are authenticated via a clientID and secret, they have to be base64 encoded and sent in the header with the parameter name as Authorisation.
Authorisation : Base64(clientID:secret)
curl --location --request GET 'https://api.sandbox.bureau.id/v2/auth/userinfo?transactionId=E1DA15EB-1111-1111-A9FA-2F1F65A9D046' \
--header 'Authorization: Basic MzNjMDBg0YzZWM3NTI1OWNiOA=='
curl --location --request GET 'https://api.bureau.id/v2/auth/userinfo?transactionId=E1DA15EB-1111-1111-A9FA-2F1F65A9D046' \
--header 'Authorization: Basic MzNjMDBg0YzZWM3NTI1OWNiOA=='
API Response
Bureau's Backend API will return one of the following HTTP status codes for every request:
{
"mobileNumber": "919932403339",
"country_code": "IN",
"status": "Success",
"authenticatedAt": "1704364405"
}
{
"statusCode": 400,
"error": {
"code": 0,
"type": "BAD_REQUEST",
"message": "Session key is missing",
"description": "request does not contain additionalData.sessionKey param in request",
"referenceId": "24f94ae8-xxxx-48a4-xxxx-b25f99fb06d9",
"metadata": null
},
"timestamp": 1658402143450,
"merchantId": "auth0|61dfbbxxxx3420071be7021",
"requestId": "66403193-xxxx-44bc-xxxx-14735a45dfeb"
}
{
"data": null,
"errors": {
"status": 401,
"errorCode": "UNAUTHORIZED",
"service": "Overwatch"
},
"message": "",
"meta": {
"length": 0,
"took": 0,
"total": 0
}
}
{
"error": {
"code": 422,
"description": "Failed to find fingerprint for given session key",
"message": "NO_RECORD_FOUND",
"metadata": null,
"referenceId": "",
"type": "NO_RECORD_FOUND"
},
"merchantId": "auth0|61dfbbxxxx420071be7021",
"requestId": "24e1aa7f-xxxx-404d-xxxx-5f8a0227e8f0",
"statusCode": 422,
"timestamp": 1658402132141
}
{
"error": {
"code": 0,
"description": "",
"message": "Server encountered an error",
"metadata": null,
"referenceId": "86529a18-a5cb-4da9-91b0-8d04cdb9167e",
"type": "INTERNAL_SERVER_ERROR"
},
"merchantId": "auth0|61dfxxxx0071be7021",
"requestId": "c69d86f0-xxxx-4ef0-xxxx-e687d595a507",
"statusCode": 500,
"timestamp": 1657009043753
}
Updated about 2 months ago