Web SDK
This SDK has the capabilities related to device intelligence and behavioural biometrics
Getting Started
This is compatible with all front-end browser frameworks with JS and HTML. The common ones include - JS, React, Vue, Flutter, and Angular.
Integration Steps
Include the following script in your HTML page.
<script src="https://fingerprint.app.stg.bureau.id/index.js"></script>
<script src="https://fingerprint.app.bureau.id/index.js"></script>
Flow Diagram
/The diagram is a typical implementation of our browser SDK.
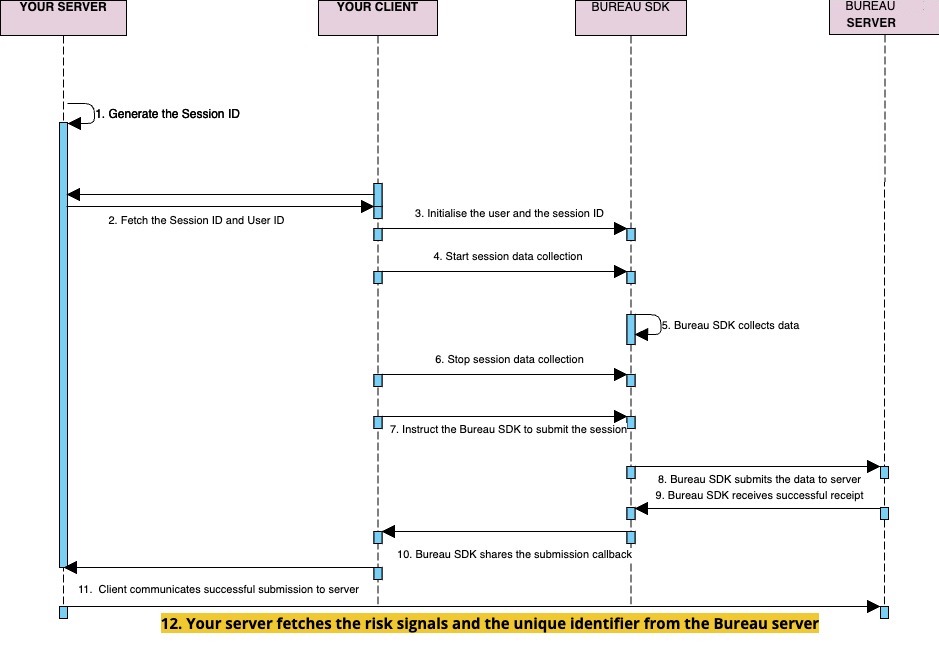
sessionID
anduserId
is made available to your front-end application (preferable from the backend where required).- Call the
BureauAPI.getBureauApiInstance()
function with the parameters ( need to call this initiate inonCreate()
of application class)- sessionId - MANDATORY
- clientId - MANDATORY
- environment - MANDATORY - default is production
- If you want to collect behavioral signals, then do the following-
- Call
startSubSession
to commence the collection of raw behavioral data points - Call
stopSubSession
to end the collection of raw behavioral data points - Ideally, a typical flow would start collecting after the launch of the application and stop collecting after the login or sign-in button is pressed when the user enters the username and password
- Call
- Call the setters functions for the below mandatory parameters
- UserID -
setUserId
- meant to be unique to a user e.g. hashed value of a PAN number or SSN or hash of mobile number
- UserID -
- Finally, call the submit function to send the device and behavioral datapoints for processing
- Call
submit
function
- Call
- Upon successful submission of the parameters, a callback is received in the SDK. The next steps can be taken based on the callback (success/failure)
- After receiving a successful callback, invoke the Bureau's backend API
/v2/services/behavioural-device-insights
to fetch the results of behavioral and device intelligence results such as device fingerprint ID, user similarity score, bot score and so on
Step 1: Initializing an SDK instance
Inside your JavaScript code, initialize SDK with the Session ID, Client ID, User ID and Environment once the page is loaded. This initializes the data-capturing functionality and collects browser data such as the device's IP address, browser, and operating system.
Example:
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId:'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'SANDBOX'
})
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId:'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'PRODUCTION'
});
Step 2: Initializing behavioral data collection
Start Session
After the SDK initialization, this starts collecting the user behavioral data like keystroke data, click data, motion data, and sensor data. Using these data points, the user similarity behavior, behavioral anomaly score and bot prediction score are calculated. To train the model on each user, six sessions are needed.
Example:
window._Fingerprint.startSession();
window._Fingerprint.startSession();
Stop Session
This stops collecting the behavioral data.
Example:
window._Fingerprint.stopSession();
window._Fingerprint.stopSession();
Note: Ideal Flow is to start collecting after the launch of the application and stop collecting after the login or sign-in button is pressed.
Step 3: Submitting an SDK
This submits the collected data to the Bureau. Invoke this method at the end of your page.
window._Fingerprint.onSubmit((response)=>{
//do something
});
Step 4: Invoke API for Insights
To access insights about users, devices, browser fingerprints, and risk signals, invoke the following API from your backend.
Sandbox - https://api.sandbox.bureau.id/v1/suppliers/device-fingerprint
Production - https://api.bureau.id/v1/suppliers/device-fingerprint
Example:
curl --location 'https://api.sandbox.bureau.id/v1/suppliers/device-fingerprint' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic MW==' \
--data '{
"sessionId": "f3199e64-cce9-47a2-a79c-67d55314"
}'
curl --location 'https://api.bureau.id/v1/suppliers/device-fingerprint' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic MW==' \
--data '{
"sessionId": "f3199e64-cce9-47a2-a79c-67d55314"
}'
Note
Ensure the Init and Submit methods are called before invoking this.
Sample integration code for login page use case
For SANDBOX:
Note: The HTML page should have basic HTML semantics with a body tag.
<html lang="en">
<head>
<meta charset="utf-8" />
</head>
<body>
<h2>Login</h2>
<form id="loginForm">
<div>
<label for="username">Username:</label>
<input type="text" id="username" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" required>
</div>
<button type="submit">Submit</button>
</form>
</body>
<script src="https://fingerprint.app.stg.bureau.id/index.js"></script>
window._Fingerprint.init({
// SDk initialization
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e3344115-7890-4238-a123-ab4bb6d',
userId:'7a68c98e-feb5-4dc0-b9ff-85b4695',
environment:'SANDBOX'
});
window._Fingerprint.startSession();
//Start collecting behavioural data
document.getElementById('loginForm').addEventListener('submit', function(event) {
event.preventDefault();
window._Fingerprint.stopSession();
//Stop collecting behavioural data
window._Fingerprint.onSubmit((res)=>{
// SDK submit call
//Make GET call to get session insights
});
});
Additional information
init
- This method integrates the SDK with an object that has an environment, session ID and client ID.
- We recommend initializing the SDK at the root of your website, but it can be placed anywhere depending on your use case.
Example:
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId :'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'SANDBOX'
});
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId :'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'PRODUCTION'
});
Parameters
sessionId
String Required
Session ID for the page. Make sure that for each init call, the sessionId must be unique. In case of duplicate sessionId, a 409 will be thrown during the submit call.
clientId
String Required
To identify the client. This will be shared with you from the Bureau. This will be a static key.
userId
String Optional
To identify the user. This can be set later using setUserId API.
environment
String Required
Run the SDK in SANDBOX or PRODUCTION mode.
Return value
Null
submit
- Invoking this function will call the Bureau's SDK POST endpoint, passing the details captured after the init() function to generate a fingerprint.
- Call this method at the end of your page.
- Examples:
- After Sign in/Sign up
- After address change confirmation
- Nominee change confirmation
- Email / password change confirmation
- After payment / pre-payment initiation
Example:
window._Fingerprint.onSubmit((response)=>{
//do something
});
Parameters
callback
Function Optional
You will get a callback on successful submission if a callback function is provided.
Example:
function callback(response){
if(response.status === 200){
console.log("Submit is successful")
}else{
console.log("Submit is not successful")
}
}
window._Fingerprint.submit(callback);
setuserid
You can also set the user ID later using the following API. Note: This should be called before 'submit API' call.
window._Fingerprint.setUserId('Merchant_ABC');
Invoke API for insights
To access insights from users and devices, including device fingerprint, and risk signals, integrating with Bureau's backend API for Device Intelligence insights.
Sample Request and Response
Below is a sample request and response for our Device Intelligence API. Refer to our Device Intelligence API documentation for more details.
Contact our support team at [email protected] to get your API keys and the production endpoint for the API.
Note
To access insights about users, devices, browser fingerprints, and risk signals, ensure the
init
andsubmit
methods are called before invoking calling the Insights API.
curl --location 'https://api.sandbox.bureau.id/v1/suppliers/device-fingerprint' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic MW==' \
--data '{
"sessionId": "f3199e64-cce9-47a2-a79c-67d55314"
}'
{
"sessionId": "0be8c4ab-1185-4b50-90ce-c5d3c3c2743a",
"userId": "",
"fingerprint": "b1630855-f27b-4ab7-ba96-bc822db36284",
"networkInformation": {
"isp": "Bharat Sanchar Nigam Limited",
"ipType": "HOME",
},
"GPSLocation": {
"city": "",
"country": "",
"latitude": 0,
"longitude": 0,
"region": ""
},
"IPLocation": {
"city": "Uttukkuli",
"country": "India",
"latitude": 11.16667,
"longitude": 77.433327,
"region": "Tamil Nadu"
},
"IPSecurity": {
"isCrawler": false,
"isProxy": false,
"isTor": false,
"VPN": false,
"threatLevel": "LOW"
},
"totalUniqueUserId": 1,
"firstSeenDays": 6,
"createdAt": 1695294348960,
"os": "",
"riskLevel": "MEDIUM",
"riskScore": 26.29,
"platform": "web",
"fingerprintConfidenceScore": "87",
"ip": "117.193.212.120",
"trueUserAgent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/116.0.0.0 Safari/537.36",
"userAgentSpoof": false,
"incognitoDetected": false,
"adBlockerDetected": false,
"botDetectionScore": 100,
"userSimilarityScore": 76.23,
"behaviouralAnomalyscore": 12.2,
"behaviouralFeatures": {
"copyPasteActivity": "LOW",
"autofillActivity": "LOW",
"swipeActivityDetected": false,
"contextSwitchActivity": true,
"fieldFocusActivity": "LOW",
"sessionDurationInMS": 636
},
"detectCanvasSpoof": false,
"anonymisationAttempted": true,
"anonymisationAttemptedReasons": ["webgl spoof", "canvas spoof", "font manipulation"]
}
Updated 9 days ago