Web SDK
Welcome to our web plugin designed to seamlessly integrate with the Bureau's Device Intelligence, enhancing the functionality of mobile apps or webpages.
Getting Started
This is compatible with all front end browser frameworks that work with JS and HTML. The common one includes - JS, React, Vue, Flutter, Angular and so on.
Integration Steps
Include the following script in your HTML page.
<script src="https://fingerprint.app.stg.bureau.id/index.js"></script>
<script src="https://fingerprint.app.bureau.id/index.js"></script>
Flow Diagram
The diagram is a typical implementation of our browser SDK.
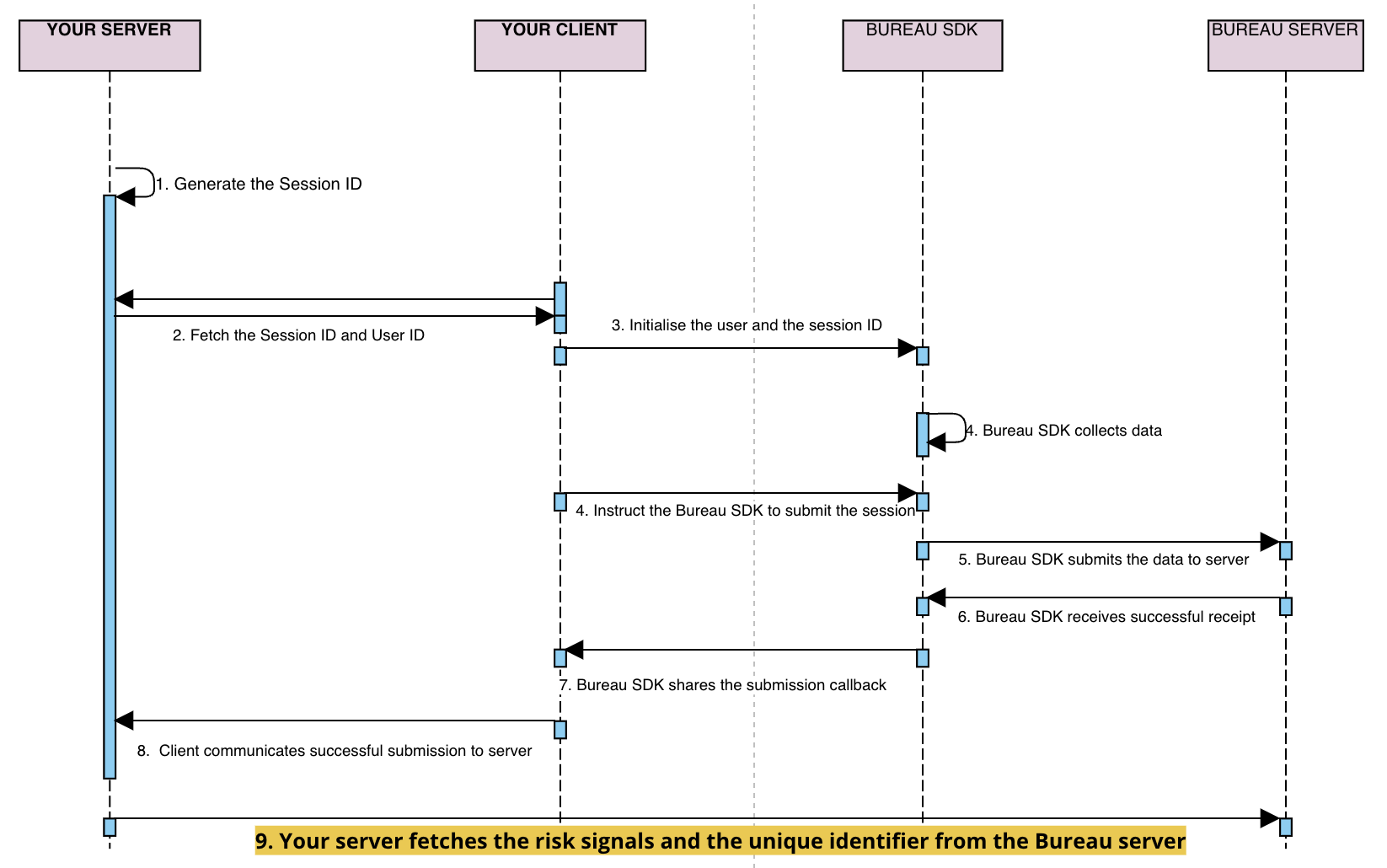
sessionKey
anduserid
are generated / retrieved by the client backend and sent to your application- Your mobile application initialises our SDK through the
init
function by setting these attributes -- Session ID (mandatory unique UUID)
- User ID (optional)
- Flow (optional)
- Invoke the
submit
function in SDK to pass the data to Bureau backend. - Upon successful submission of the parameters, a
callback
is received in the SDK. The next steps can be taken based on thecallback
(success/failure). - If the callback is successful, your mobile application relays the
success
to your backend - Invoke Bureau's backend API
/v1/suppliers/device-fingerprint
to fetch insights- Input :
sessionId
- Input :
- Based on the insights provided (fingerprint, and risk signals), you can determine the next steps for the user, such as allowing, blocking, or redirecting them.
Step 1 - Initializing an SDK instance
Inside your JavaScript code, initialize SDK with the Session ID, Client ID, and environment once the page is loaded. This initializes the data-capturing functionality and collects browser data such as the device's IP address, browser, and operating system.
Example:
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId:'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'SANDBOX'
});
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId:'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'PRODUCTION'
});
Step 2 - Submitting an SDK
This submits the collected data to the Bureau and generates a fingerprint. Invoke this method at the end of your page.
window._Fingerprint.onSubmit((response)=>{
//do something
});
Step 3 - Invoke API for Insights
To access insights from users and devices, including device fingerprint, and risk signals, integrating with Bureau's backend API for Device Intelligence insights.
Sample Request and Response
Below is a sample request and response for our Device Intelligence API. Refer to our Device Intelligence API documentation for more details.
Contact our support team at [email protected] to get your API keys and the production endpoint for the API.
curl --location --request POST 'https://api.overwatch.dev.bureau.id/v1/suppliers/device-fingerprint' \
--header 'Authorization: Basic MzNiNxxxx2ItZGU2M==' \
--header 'Content-Type: application/json' \
--data-raw '{
"sessionKey": "697bb2d6-1111-1111-1111-548d6a809360"
}'
{
"networkInformation": {
"isp": "Atria Convergence Technologies Pvt. Ltd.",
"ipType": "HOME"
},
"GPSLocation": {
"city": "Madurai",
"country": "India",
"latitude": 9.944328308105469,
"longitude": 78.12307672582392,
"region": "TN"
},
"IP": "106.51.24.95",
"IPType": "v4",
"IPLocation": {
"city": "Bengaluru",
"country": "India",
"latitude": 12.976229667663574,
"longitude": 77.60328674316406,
"region": "Karnataka"
},
"IPSecurity": {
"is_crawler": false,
"is_proxy": false,
"is_tor": false,
"threat_level": "LOW",
"VPN": false
},
"OS": "ios",
"debuggable": true,
"emulator": false,
"fingerprint": "8387e7df-320f-4b53-83c8-424ee99ea033",
"firstSeenDays": 90,
"mockgps": false,
"model": "D53gAP",
"package": "com.bureau.deviceBB",
"jailbreak": false,
"userId": "hariharaprabu",
"totalUniqueUserId": 1,
"remoteDesktop": false,
"sessionId": "Demo-5527D044-D8CF-4DC8-9351-4A0242CBF25F",
"createdAt": 1715231667924,
"confidenceScore": 85.71428571428571,
"voiceCallDetected": false,
"riskScore": 85.57,
"riskLevel": "VERY_HIGH",
"riskCauses": [
"DEBUGGER",
"DEVELOPER_MODE",
"NOT_INSTALLED_FROM_APP_STORE"
],
"developerMode": true,
"appStoreInstall": false,
"fridaDetected": false,
"accessibilityEnabled": false,
"mitmAttackDetected": false,
"behaviouralRiskLevel": "UNKNOWN",
"deviceRiskScore": 85.57,
"deviceRiskLevel": "VERY_HIGH"
}
Note: Make sure the Init and Submit methods are called before invoking this.
Learn more
Sample integration code for login page use case
For SANDBOX:
Note: The HTML page should have basic HTML semantics with a body tag.
<html lang="en">
<head>
<meta charset="utf-8" />
</head>
<body>
<h2>Login</h2>
<form id="loginForm">
<div>
<label for="username">Username:</label>
<input type="text" id="username" required>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" required>
</div>
<button type="submit">Submit</button>
</form>
</body>
<script src="https://fingerprint.app.stg.bureau.id/index.js"></script>
window._Fingerprint.init({
// SDk initialization
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e3344115-7890-4238-a123-ab4bb6d',
userId:'7a68c98e-feb5-4dc0-b9ff-85b4695',
environment:'SANDBOX'
});
document.getElementById('loginForm').addEventListener('submit', function(event) {
event.preventDefault();
window._Fingerprint.onSubmit((res)=>{
// SDK submit call
//Make GET call to get session insights
});
});
Additional information
init
- This method integrates the SDK with an object that has an environment, session ID and client ID.
- We recommend initializing the SDK at the root of your website, but it can be placed anywhere depending on your use case.
Example:
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId :'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'SANDBOX'
});
window._Fingerprint.init({
sessionId:'f3199e64-cce9-47a2-a79c-67d55314',
clientId:'3e912115-7890-4238-a123-ab4bb6d82975',
userId :'7a68c98e-feb5-4dc0-b9ff-85b469ba97b5',
environment:'PRODUCTION'
});
Parameters
sessionId
String Required
Session ID for the page. Make sure that for each init call, the sessionId must be unique. In case of duplicate sessionId, a 409 will be thrown during the submit call.
clientId
String Required
To identify the client. This will be shared with you from the Bureau. This will be a static key.
userId
String Optional
To identify the user. This can be set later using setUserId API.
environment
String Required
Run the SDK in SANDBOX or PRODUCTION mode.
Return value
Null
submit
- Invoking this function will call the Bureau's SDK POST endpoint, passing the details captured after the init() function to generate a fingerprint.
- Call this method at the end of your page.
- Examples:
- After Sign in/Sign up
- After address change confirmation
- Nominee change confirmation
- Email / password change confirmation
- After payment / pre-payment initiation
Example:
window._Fingerprint.onSubmit((response)=>{
//do something
});
Parameters
callback
Function Optional
You will get a callback on successful submission if a callback function is provided.
Example:
function callback(response){
if(response.status === 200){
console.log("Submit is successful")
}else{
console.log("Submit is not successful")
}
}
window._Fingerprint.submit(callback);
setuserid
You can also set the user ID later using the following API. Note: This should be called before 'submit API' call.
window._Fingerprint.setUserId('Merchant_ABC');
Invoke API for insights
To access insights from users and devices, including device fingerprint, and risk signals, integrating with Bureau's backend API for Device Intelligence insights.
Sample Request and Response
Below is a sample request and response for our Device Intelligence API. Refer to our Device Intelligence API documentation for more details.
Contact our support team at [email protected] to get your API keys and the production endpoint for the API.
Note
To access insights about users, devices, browser fingerprints, and risk signals, ensure the
init
andsubmit
methods are called before invoking the Insights API.
curl --location 'https://api.sandbox.bureau.id/v1/suppliers/device-fingerprint' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic MW==' \
--data '{
"sessionId": "f3199e64-cce9-47a2-a79c-67d55314"
}'
{
"sessionId": "0be8c4ab-1185-4b50-90ce-c5d3c3c2743a",
"userId": "",
"fingerprint": "b1630855-f27b-4ab7-ba96-bc822db36284",
"networkInformation": {
"isp": "Bharat Sanchar Nigam Limited",
"ipType": "HOME"
},
"GPSLocation": {
"city": "Bangalore",
"country": "India",
"latitude": 51.5207,
"longitude": -0.1550,
"region": "Karnataka"
},
"IPLocation": {
"city": "Bangalore",
"country": "India",
"latitude": 51.5207,
"longitude": -0.1550,
"region": "Karnataka"
},
"IPSecurity": {
"isCrawler": false,
"isProxy": false,
"isTor": false,
"VPN": false,
"threatLevel": "LOW"
},
"totalUniqueUserId": 1,
"firstSeenDays": 6,
"createdAt": 1695294348960,
"OS": "",
"riskLevel": "MEDIUM",
"riskScore": 26.29,
"platform": "web",
"fingerprintConfidenceScore": "87",
"ip": "192.168.369.619",
"trueUserAgent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/116.0.0.0 Safari/537.36",
"userAgentSpoof": false,
"incognitoDetected": false,
"adBlockerDetected": false,
"botDetectionScore": 100,
"detectCanvasSpoof": false,
"anonymisationAttempted": true,
"anonymisationAttemptedReasons": [
"webgl spoof",
"canvas spoof",
"font manipulation"
]
}
Updated 5 days ago
Understand how to fetch insights from the server side endpoint